Who Should Take This Class: Anyone who wants to learn how digital media are created and manipulated. Ever wondered how Photoshop filters work, or how a keyboard can make trumpet and dog sounds, or how people can be made to disappear in videos? That’s what we’ll explain, and you’ll make them yourself.
You do not have to be a programmer! You don’t have to want to be a programmer! You are expected to have created and executed a program (even in Scratch, even in high school) at some point in the past.
Programming in Python: The kind of programs we make will be a lot like those in COMPFOR 121, but in Python. We are planning to use Thonny, the easiest to install and easiest to use Python IDE (Integrated Development Environment) we can find. We expect no one to have to use a command line. It will work for Windows, Macintosh, or Linux, but not Chromebook.
Below is an example of using Thonny to implement a picture filter that increases red in a picture. The code looks like this:
def increaseRed(p):
for px in getPixels(p):
setRed(px,2*getRed(px))
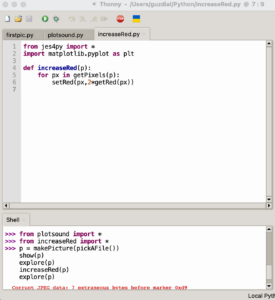
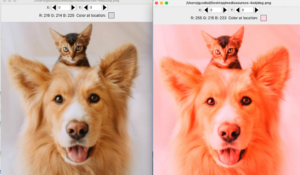
To edit sounds, we change individual samples in a sound. Here’s a piece of Python code that increases the volume in the first half of the sound.
from jes4py import *
def increaseVolumeFirstHalf(sound):
samples = getSamples(sound)
for i in range(0,int(len(samples)/2)):
v = getSampleValueAt(sound,i)
setSampleValueAt(sound,i, 2 * v)
Course Goals
At the end of this class students will be able to:
- Create and design software for manipulating and creating digital media.
- Be able to describe how text, images, sounds, and video are represented on the computer.
- Be able to write Python programs that transform text, images, and sounds through filters.
- Be able to write Python programs that generate text, images, sounds, and videos.
- Be able to explain what an object is in object-oriented programming, and use objects to define digital media
Homework and Projects: Students will write 5-6 Python programs for homework and one final project. Expected homework:
- Encode and decode text messages
- Create image filters and a collage of your own pictures manipulated with your own filters.
- Create a sound collage of several sounds spliced together with digital manipulations
- Create a video based on an image effect
Expected Grading
- 35% – Five Homework Assignments in Python turned in via Canvas
- 25% – Seven online quizzes. (There will be eight quizzes, lowest score will be dropped. They are released on Wednesday and due on Sunday.)
- 20% – Final Project. Students will be asked to create a video with both visual and audio components of at least 30 seconds in length, with all elements generated through their programs.
- 20% – Participation (in-class activities and on-line forum). Typically, 90% attendance gives you 100% of participation points, 80% gives you 90% of participation points, and so on.
There will be no midterm or final exams.
Media Computation
This course is a kind of “Media Computation” course. Originally developed at Georgia Tech in the early 2000’s, it’s been successfully taught all over the world. Here are some examples of Media Computation in videos: